Good screen management is not a matter of luck, but rather the right choice of suitable solutions within the Qt 6 application. This allows you to achieve a smooth and efficient user experience. Whether you are developing a simple app with a few screens or a complex application with dynamic interfaces, understanding the different methods available in Qt 6 will help you make informed decisions. In this article, I’ll introduce various screen management techniques and go over their implementations, pros and cons, and the scenarios in which each method shines.
1. Dynamic screen creation with “component.createComponent” and “component.createObject”
One of the powerful features of Qt 6 is the ability to create components dynamically at runtime. This is particularly useful when the number or type of screens is not known at compile time.
1.1 Practical example```qml
import QtQuick 2.15
import QtQuick.Controls 2.15
ApplicationWindow {
visible: true
width: 640
height: 480
Button {
text: "Create Screen"
onClicked: createScreen()
}
function createScreen() {
var component = Qt.createComponent("Screen.qml");
if (component.status === Component.Ready) {
var dynamicScreen =
component.createObject(applicationWindow);
dynamicScreen.width = 640;
dynamicScreen.height = 480;
}
}
function destroyScreen(screen) {
if (screen !== null) {
screen.destroy();
}
}
}
In this example, a new screen is created dynamically when the button is clicked. The function `Qt.createComponent` loads the QML component and `createObject` realizes it.
1.3 Advantages and disadvantages of dynamic screen creation
Dynamic creation offers flexibility and efficient memory usage as components are only displayed when needed. However, there may be a slight delay during creation which requires careful error handling to manage component load status and potential errors. This method is ideal for scenarios where screens are not required simultaneously, such as in multi-level forms or feature-rich applications with many optional modules.
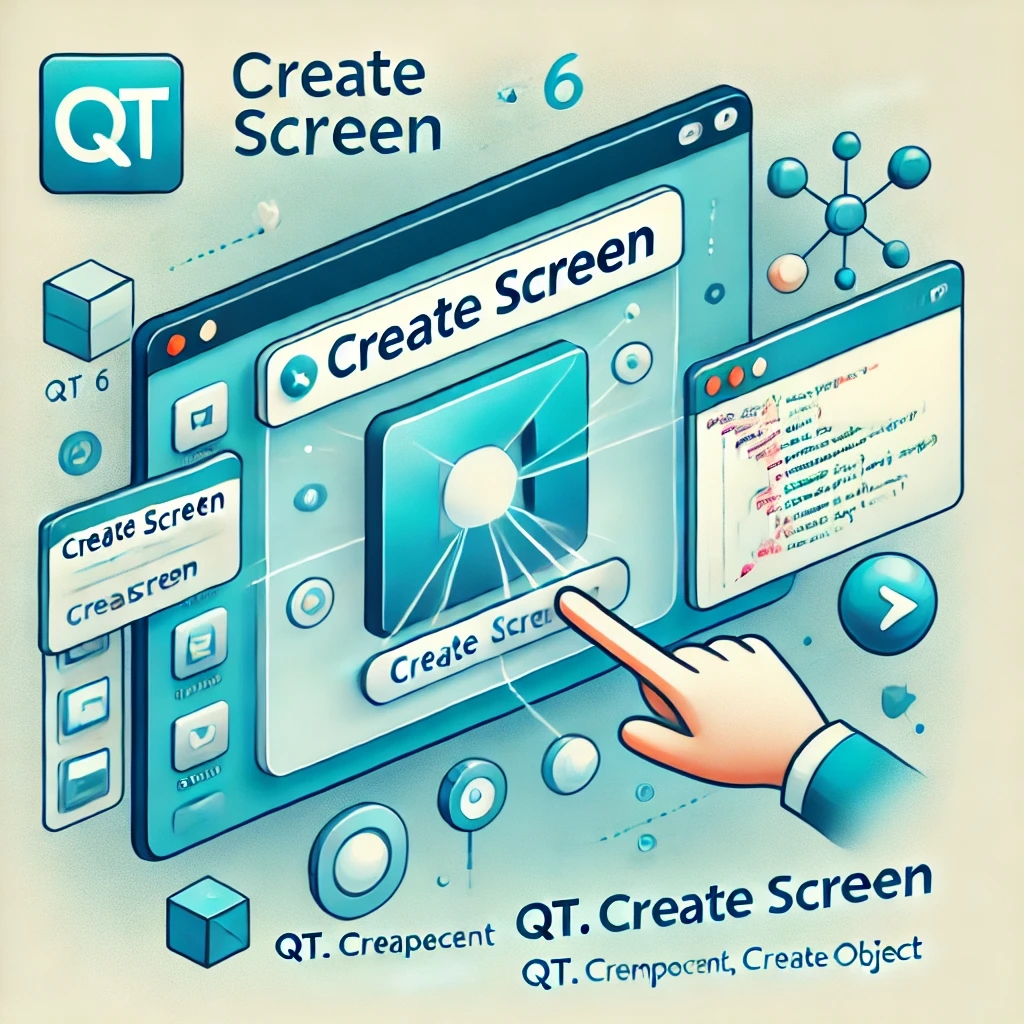
1.4 Further reading
– Dynamic QML object creation with JavaScript: https://doc.qt.io/qt-6/qtqml-javascript-dynamicobjectcreation.html
2. Screen management with `Loader`
The “Loader” element in Qt 6 provides a convenient way to manage the loading and unloading of QML components, enabling lazy loading and reducing the initial memory footprint of your application.
2.1 Practical example```qml
import QtQuick 2.15
import QtQuick.Controls 2.15
ApplicationWindow {
visible: true
width: 640
height: 480
Loader {
id: screenLoader
anchors.fill: parent
}
Button {
text: "Load Screen"
onClicked: screenLoader.source = "Screen.qml"
}
}
Here a `loader` is used to load a screen when the button is clicked. The `loader` can also unload the component by setting its `source` property to an empty string.
2.2 Advantages and disadvantages of the loader in Qt 6
Using `Loader` simplifies memory management and improves performance by loading components only when needed. However, frequent loading and unloading can lead to performance bottlenecks, especially if the components are complex. Loader is best suited to applications where screens are used intermittently and do not need to be held in memory when they are no longer visible.
2.3 Further reading
– Qt documentation for the loader: https://doc.qt.io/qt-6/qml-qtquick-loader.html
– Lazy loading in Qt Quick: https://doc.qt.io/qt-6/qtquick-performance.html#lazy-loading
3. Screen change based on visibility
Another simple approach is to create all screens at once and manage their visibility with the “visible” property.
3.1 Practical example
```qml
import QtQuick 2.15
import QtQuick.Controls 2.15
ApplicationWindow {
visible: true
width: 640
height: 480
Rectangle {
id: screen1
widt
h: parent.width
height: parent.height
color: "red"
visible: true
}
Rectangle {
id: screen2
width: parent.width
height: parent.height
color: "blue"
visible: false
}
Button {
text: "Switch Screen"
onClicked: {
screen1.visible = !screen1.visible;
screen2.visible = !screen2.visible;
}
}
}
In this example, two screens are created and their visibility is switched by clicking on a button.
3.2 Advantages and disadvantages of the visible property
The management of screens with visibility is simple and quick to implement. However, it consumes more memory as all screens are always kept in memory, which can be a disadvantage for applications with many screens or resource-intensive components.
This approach is suitable for small to medium-sized applications where the effort required to keep all screens in memory is negligible.
3.3 Further reading
– Qt documentation for property binding: https://doc.qt.io/qt-6/qtqml-syntax-propertybinding.html
4. Stack-based navigation with “StackView”
“StackView” offers a stack-based navigation model in which screens are pushed and pulled out of the stack. This is able to imitate a typical navigation pattern and can be found primarily in mobile applications.
4.1 Practical example
```qml
import QtQuick 2.15
import QtQuick.Controls 2.15
ApplicationWindow {
visible: true
width: 640
height: 480
StackView {
id: stackView
anchors.fill: parent
initialItem: Screen1 {}
}
Button {
text: "Push Screen"
onClicked: stackView.push(Qt.resolvedUrl("Screen2.qml"))
}
}
```
Here, a `StackView` manages the navigation between the screens. The `initialItem` property sets the first screen and additional screens are pushed onto the stack using the `push` method.
4.2 Advantages and disadvantages of StackView
“StackView” provides an intuitive way to manage navigation and maintains a clear screen history, which is useful for back navigation. However, managing complex navigation stacks can become cumbersome and affect performance if not handled correctly.
This method is recommended for applications with hierarchical navigation structures, such as settings menus or multi-level processes where back navigation is required.
4.3 Further reading
– Qt documentation for StackView: https://doc.qt.io/qt-6/qml-qtquick-controls-stackview.html
– Implementation of navigation with StackView: https://doc.qt.io/qt-6/qtquickcontrols2-navigation.html
5. Swipe-based navigation with “SwipeView”
“SwipeView” offers a touch-friendly navigation model, ideal for mobile applications or touch interfaces where users can swipe between different screens.
5.1 Practical example
```qml
import QtQuick 2.15
import QtQuick.Controls 2.15
ApplicationWindow {
visible: true
width: 640
height: 480
SwipeView {
anchors.fill: parent
Rectangle { color: "red"; width: parent.width; height:
parent.height }
Rectangle { color: "blue"; width: parent.width; height:
parent.height }
Rectangle { color: "green"; width: parent.width; height:
parent.height }
}
}
In this example, “SwipeView” is used to allow users to swipe between three different screens, which are displayed as “rectangle” components with different colors.
5.3 Further reading
– Qt documentation for SwipeView: https://doc.qt.io/qt-6/qml-qtquick-controls-swipeview.html
6. Tab-based navigation with “TabBar”
“TabBar” provides a tabbed navigation model that allows users to switch between different tabs, each representing a different screen or section of the application.
6.1 Practical example
import QtQuick 2.15
import QtQuick.Controls 2.15
ApplicationWindow {
visible: true
width: 640
height: 480
TabBar {
id: tabBar
width: parent.width
TabButton {
text: "Red"
onClicked:
stackView.replace(Qt.resolvedUrl("RedScreen.qml"))
}
TabButton {
text: "Blue"
onClicked:
stackView.replace(Qt.resolvedUrl("BlueScreen.qml"))
}
TabButton {
text: "Green"
onClicked:
stackView.replace(Qt.resolvedUrl("GreenScreen.qml"))
}
}
StackView {
id: stackView
anchors.top: tabBar.bottom
anchors.left: parent.left
anchors.right: parent.right
anchors.bottom: parent.bottom
initialItem: RedScreen {}
}
}
In this example, ‘TabBar’ is used in conjunction with ‘StackView’ to create a tabbed interface. Each tab button replaces the current screen in ‘StackView’ with a new one.
6.2 Advantages and disadvantages of TabBar
TabBar provides a familiar and easy to use navigation model and is therefore ideal for desktop applications or applications with clearly defined sections. However, it may not be as suitable for applications with a large number of screens or those that require a more dynamic navigation flow. TabBar is perfect for settings pages, dashboards or applications with a few primary sections.
6.3 Further reading
– Qt documentation for TabBar: https://doc.qt.io/qt-6/qml-qtquick-controls-tabbar.html
– And about TabButton: https://doc.qt.io/qt-6/qml-qtquick-controls-tabbutton.html
7. Conclusion
Choosing the right solution for managing screens in Qt 6 depends on the specific requirements of your application. Dynamic creation with `component.createComponent` and `component.createObject` provides flexibility and efficiency for dynamic interfaces. Loader’ provides an easy way to manage memory usage by loading screens on demand. Visibility-based switching is quick to implement but can consume more memory. StackView is ideal for applications with hierarchical navigation. SwipeView and TabBar provide intuitive navigation models for mobile and desktop applications respectively.
Get involved with these methods. The Qt team at helloQt will be happy to help you with the implementation. Then you can create more efficient and responsive Qt 6 applications that are tailored to the individual requirements of your projects.